1.4. C++ Tips
1.4.1. lower_bound and upper_bound
STL provides binary search functions std::lower_bound and std::upper_bound.
If you have multiple elements in the range [first, last) where no value equals the value you are searching for, lower_bound and upper_bound return the same iterator. If there is value euqal to what you are searching for, there will be difference.
Assuming you want to insert a value to a sorted array, lower_bound returns the left-most position you can insert the value into, and upper_bound return the right-most position you can insert.
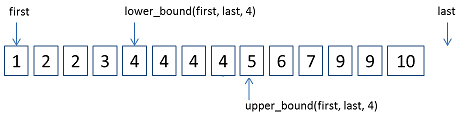
Let’s assume we use them to search for 2 in the following collections. The arrows show what iterators the two would return:
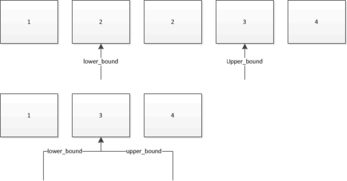
lower_bound result can be equal or greater than the given key K, but upper_bound result is always greater than K
.
In Java, floorEntry()
returns a key-value mapping associated with the greatest key less than or equal to the given key
, or null if there is no such key.
In C++’s map
, the equivalent code should be like:
auto it = prev(map.upper_bound(key));
Also, std::equal_range will return both the lower and upper bound in a pair, in a single call.
STL std::map
and std::set
have two member functions with the same names.
Note
The two functions only require the first two parameters are forward iterators and the value is comparable, so it is fine to apply to unsorted sequence if necessary.
1 const vector<int> raw = {5, 3, 4, 1};
2 lower = lower_bound(raw.begin(), raw.end(), 4);
3 EXPECT_TRUE(lower == next(raw.begin(), 2));
4 lower = lower_bound(raw.begin(), raw.end(), 6);
5 EXPECT_TRUE(lower == raw.end());
6 lower = lower_bound(raw.begin(), raw.end(), 0);
7 EXPECT_TRUE(lower == raw.begin());
Note
They can also be applied to std::list
.
1 const list<int> data = {1, 2, 2, 3, 4, 4, 4, 4, 5, 6, 7, 9, 9, 10};
2 auto lower = lower_bound(data.begin(), data.end(), 4);
3 EXPECT_TRUE(lower == next(data.begin(), 4));
Reference:
Longest Increasing Subsequence(LIS)(Section 3.4.1.19)
Question: Maximum Profit in Job Scheduling(Section 3.4.1.18)
https://www.geeksforgeeks.org/java-util-treemap-floorentry-floorkey-java/
dynamic dispatch, vpointer and virtual table: https://blog.csdn.net/luanfenlian0992/article/details/118771472
1.4.2. Virtual Function
A virtual function is a member function in the base class that we expect to redefine in derived classes.
Basically, a virtual function is used in the base class in order to ensure that the function is overridden. This especially applies to cases where a pointer of base class points to an object of a derived class.
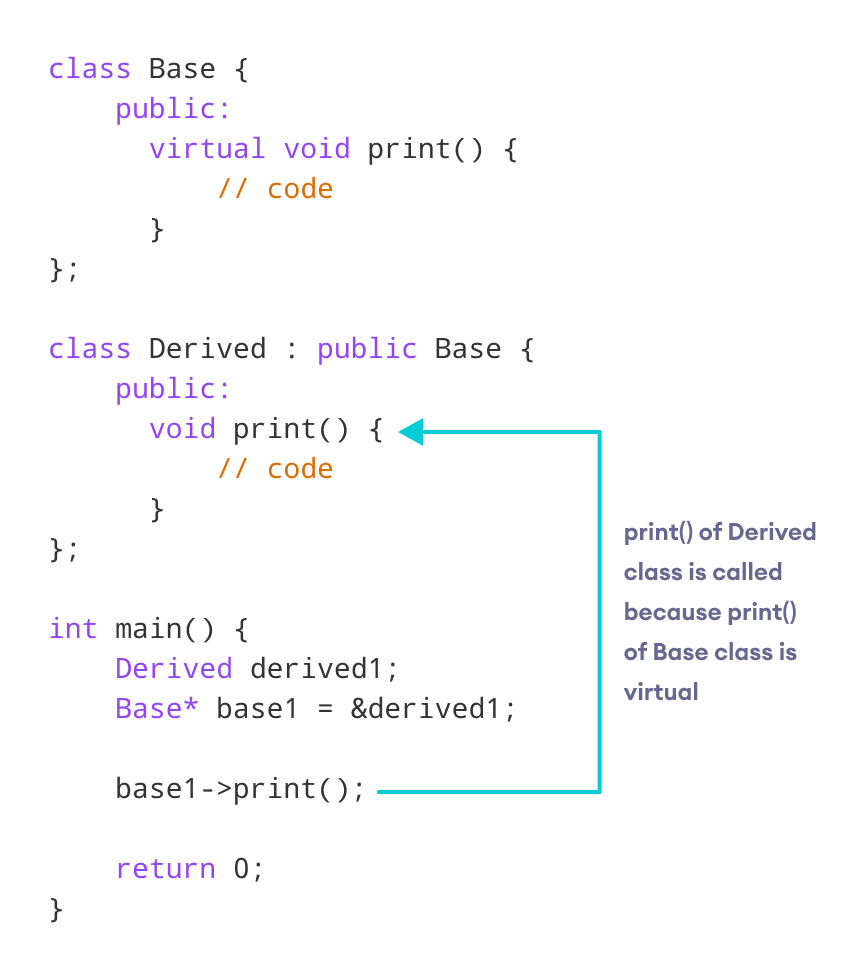